I built my first web app! 🚀 (Days 61-70: Code with Benny)
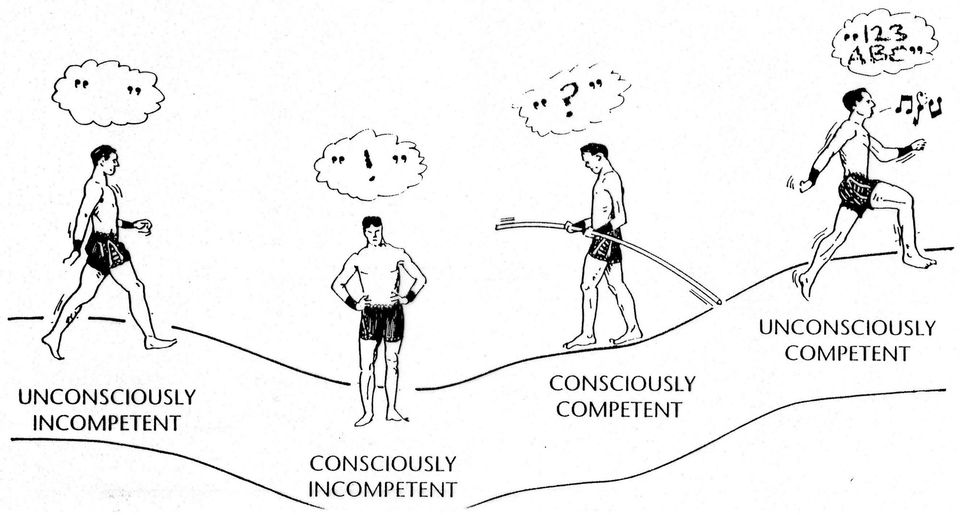
I BUILT MY FIRST WEB APP!! You can check it out here, or head over to my projects page: bennyrubanov.com/projects.
It tells you if you should read the news today or not based on how negative the news is, and your tolerance of bad news.
You can see the code here (on replit) or on github (current deployed branch is V2).
And if you didn't catch it in my last email(s): I launched my YouTube channel! I’ve uploaded a video version of this article there (also, see below). Like/comment/subscribe: all your support is very appreciated ♥️
I also uploaded a couple more TikToks - check them out on my page: tiktok.com/@bennyrubanov.
Quick statistics:
- V1 took 16-20 hours to build, spread over ~3.5 days
- V2 (the currently deployed version) took around an additional 30 hours to build
- The final version, as of this writing, has ~474 lines of code
Languages used:
- Python + Flask (Flask is a micro web framework written in Python)
- HTML
- Javascript
Tools used:
- Replit (for my coding environment)
- NewsAPI
- Notion (for documenting)
- Google Cloud Translation API
- VADER sentiment analysis package
- Replicate (for V1 using Llama 2)
- ChatGPT 🙂
How I did it
and some additional thoughts :)
Table of contents:
- Learning how to learn
- Overall thoughts
- How I built it (day-by-day breakdown)
- What's next for the app
- What's next for me
Learning how to learn
You may have noticed a fun graphic at the top of this newsletter - it's one of my favorites. It depicts the concept in psychology of the “four stages of competence”, which addresses how when humans learn a new skill, they tend to progress through roughly four phases of skill-learning:
- Unconscious incompetence
- Conscious incompetence
- Conscious competence
- Unconscious competence
I think I fall mostly in #2 at the moment - I know some things, and I think I have a decent grasp broadly of knowing what I don't know. But there is a LOT of work to be done to jump over to conscious competence, and I know that it'll happen in bits and pieces across the very wide gamut of skills that comprise the umbrella of "software engineering skill".
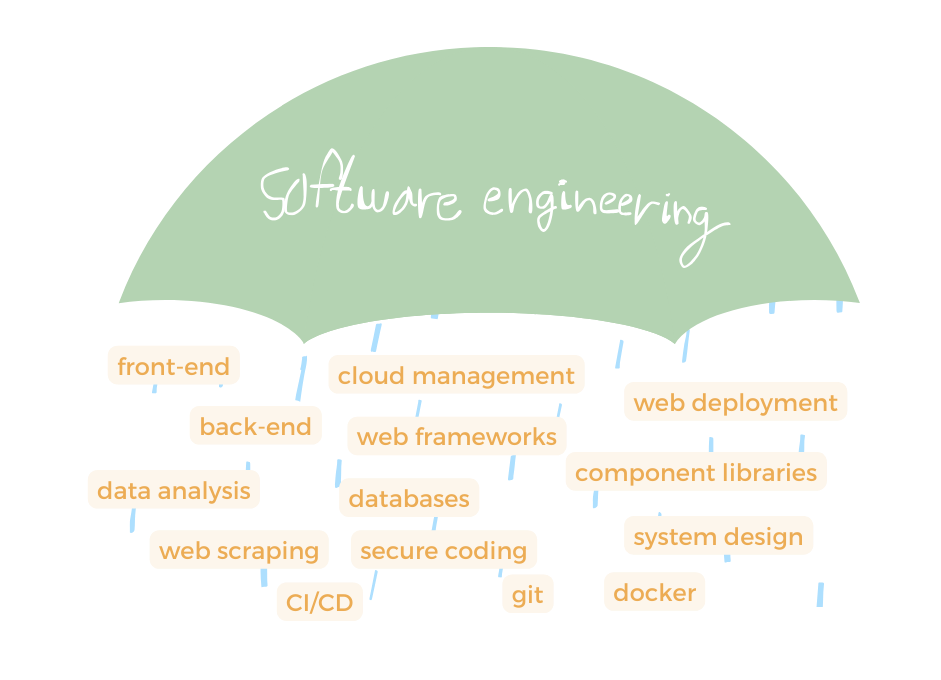
Completing this app gave me a huge confidence boost that I think was needed. To see something go from an idea in my head to something that worked from end-to-end, on its own, 24/7, was incredibly rewarding. Before this, I felt overwhelmed by how much there was to learn (as I was crossing into the “consciously incompetent” phase of learning).
All this to say: I have lots to learn, but building this web app was a big confidence boost. And most importantly: I now have a lot more confidence that I have the tools and mental faculties to be able to figure out whatever I need to figure out to build whatever I want to build. I am learning how to learn.
Some quick overall thoughts:
- While building the app, I felt something I haven’t felt in a while, but have sorely missed: hitting “flow mode”. It felt as if time melted away while I was engrossed completely in building this app. I spent many hours in nonstop flow, going back-and-forth with ChatGPT, reading API documentation, going through my learnings database to look through past learnings, researching technologies and models, and more.
- I’m really happy with how the app turned out. Even though on the front-end, it’s quite simple, I combined quite a few new skills I learned before or during building to make this thing run. It uses 4 external packages/APIs, and getting these external services to talk to each other correctly was a fun challenge.
- The first version of the app used Llama2, Meta’s newest AI model, to do the analysis, but that got a bit expensive 🙂. I have since switched to a free python sentiment analysis package (that is also much faster!)
Now, for the technical stuff. 👇
How I built it and what I learned
đź’ˇYou can see the unedited notes I took while building the app here. This is where I wrote down what I did day-to-day and the many obstacles that I came up against.
Day 1
- Today was all about figuring out the AI model (though I later on switched to the free python sentiment analysis package I mentioned above)
- I started writing the app with some code from replit 100 days of python course on combining OpenAI's API and NewsAPI:
import os, requests, json, openai
news = os.environ['news']
openai.organisation = os.environ['organisationID']
openai.api_key = os.environ['openai']
openai.Model.list()
country = "gb"
url = f"https://newsapi.org/v2/top-headlines?country={country}&apiKey={news}"
result = requests.get(url)
data = result.json()
counter = 0
for article in data["articles"]:
counter +=1
if counter > 5:
break
prompt = (f"""Summarise {article["url"]} in one sentence.""")
response = openai.Completion.create(model="text-davinci-002", prompt=prompt, temperature=0, max_tokens=50)
print(response["choices"][0]["text"].strip())
- I ran into a bunch of trouble where playing with the demo for the llama2 model at https://replicate.com/replicate/llama-2-70b was not giving me the output I wanted. Then I realized I needed something like ChatGPT, and figured somebody probably pre-trained a model for this on replicate (it took a while to get to this conclusion though, and I emailed this guy for help and posted in the replicate discord before I figured this out lol)
- I tested the APIs for the 13b param model and 70b param model and preliminarily found that they were similar in terms of quality of output, but somehow it seemed the 70b model was cheaper, so I went with that for a while
Day 2
I forgot to document all of the issues I went through this day, but there were many. This is the day that ChatGPT probably started getting annoyed with me:
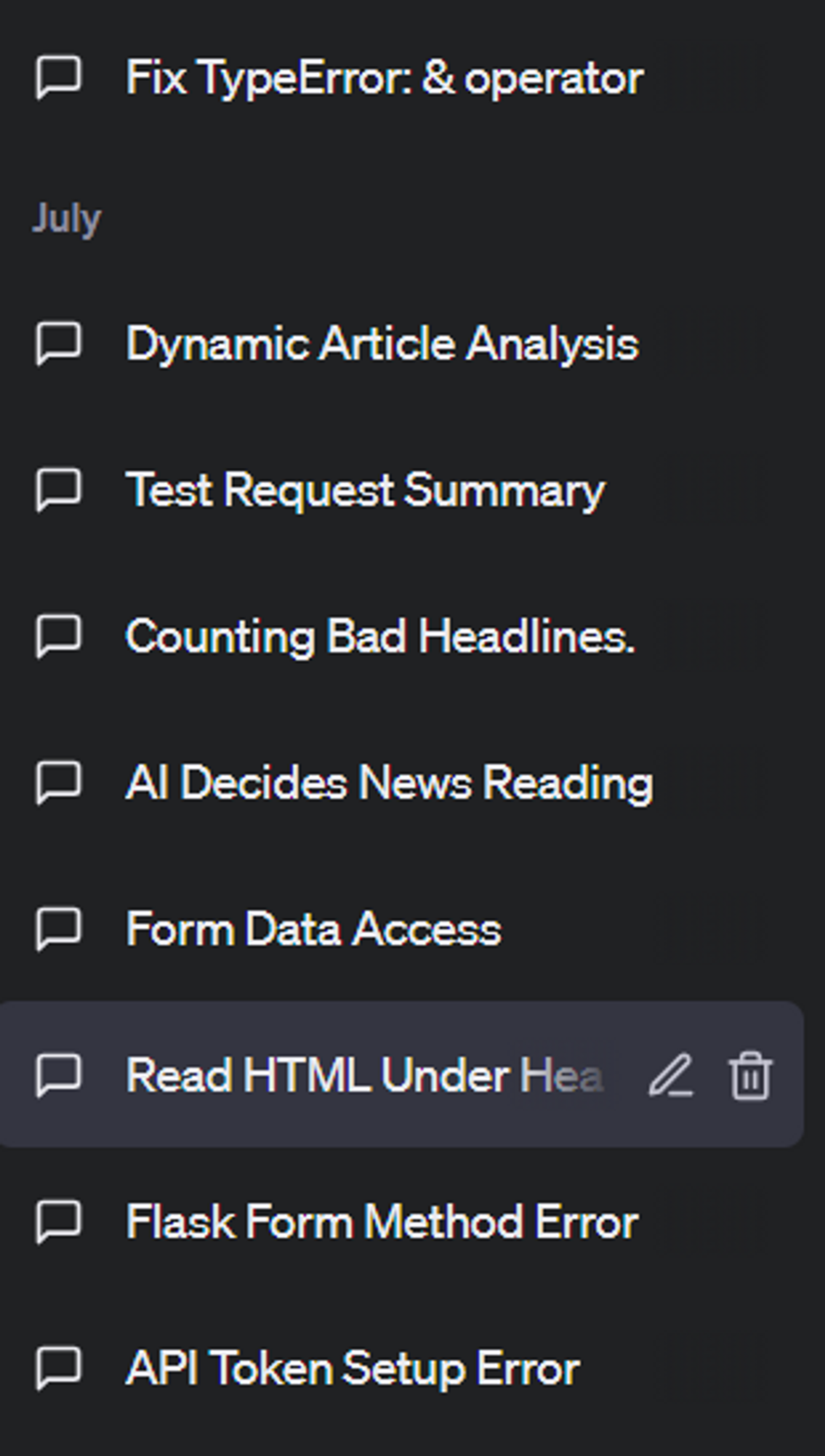
I finished the day with this code, which included:
- a simple front end for the user done with two input forms and a submit button using flask + html
- a result page to display the recommendation to the user
- the different messages depending on the user’s input and the articles
- both APIs being called, headlines being passed to the AI model effectively
Day 3
- I fixed an issue where the model I was using wasn’t giving me an output in only one of 3 forms (negative, positive, neutral). Using the “system prompt” function of the API for this model fixed this. The model: a16z-infra/llama-2-13b-chat
- Created a little "how this works" page
- Tried to tackle an issue I called “dynamic article analysis” where I wanted the articles to appear on the front end as they were being analyzed (and on the same page, rather than redirecting the user to another one). Gave it a try today but didn’t get far
- Ended up deploying the bad boy today, and this was the first time I got to see something I had created from scratch working and online 🙂
Day 4
- Tried for a LONG time to try to get dynamic article analysis to pop up. ChatGPT definitely hated me at this point: https://sharegpt.com/c/YFLsbba
- But I got it to work!!
- Broke out the code into different files as opposed to having them all in one
- Fixed some bugs
And that was essentially the end of the build process for V1. After this, I figured out how to deploy the app via Replit, sent it around to some close friends and family for some feedback, and started planning for V2 🙂
My app-building notes contain the documentation for the V2 build process as well, if you want to read on!
What’s next for the app
Primarily, I’m going to use this project as a staging ground for testing new skills I learn, with a focus on improving the front-end. Right now, of course, it’s super simple, using the most basic HTML elements with no CSS styling:
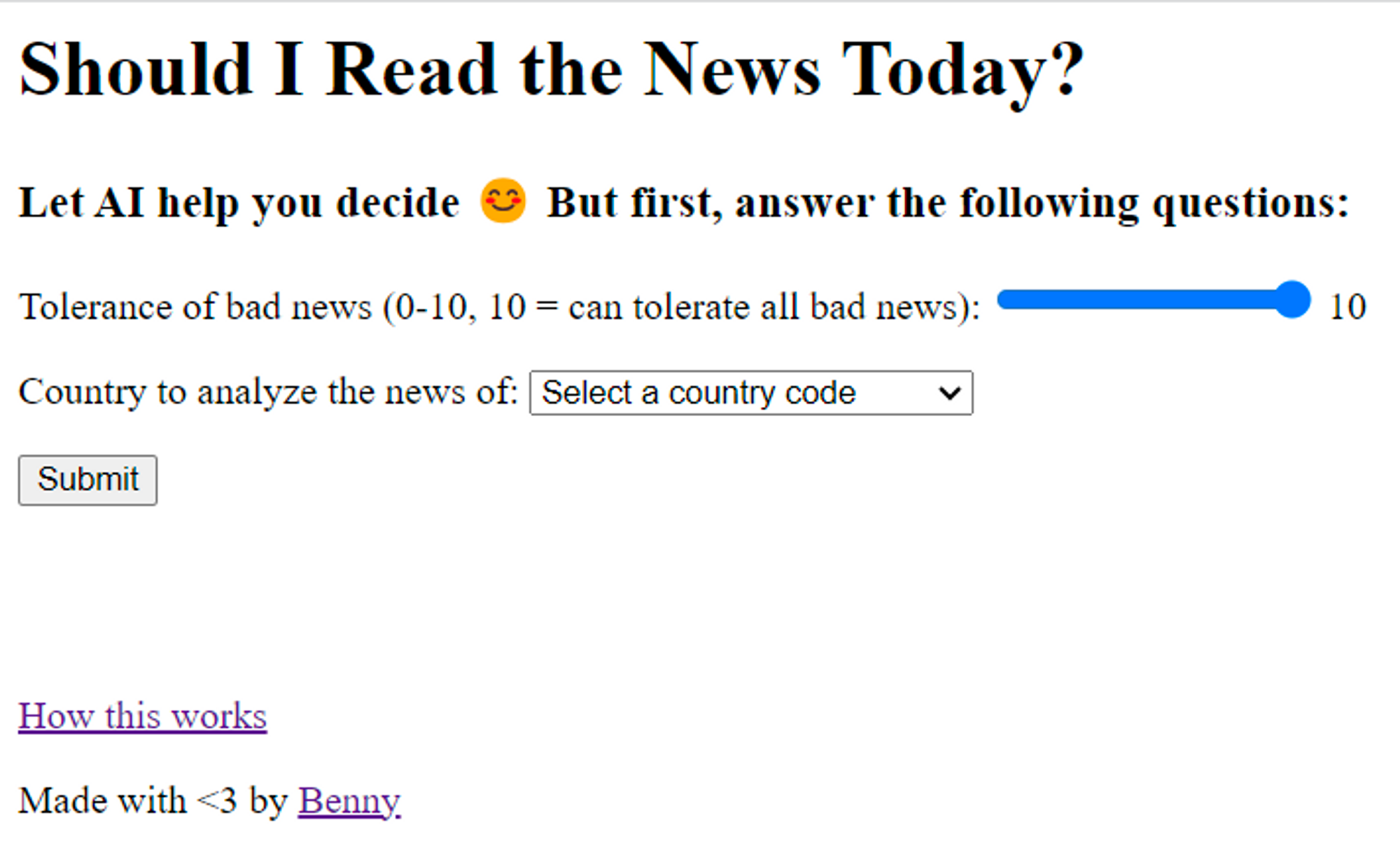
But I’m excited to learn and play around with React and component libraries like Shadcn/ui (recommended to me by a friend in Bali).
If I were to build a full V3 of this app, some ideas I’ve gotten from friends and family that intrigue me are:
- Add categories for sports, politics, etc
- Rate articles based on political leaning using AI
- Sort news from positive to negative, and maybe deliver this to users based on their preferences (or to help acclimate them to bad news in a healthy way?)
- Prove out if people read more headlines if they are less negative? Maybe provide a service to help write news more clickable/digestible news headlines for news organizations
What’s next for me?
I want to finish my 100 Days of Coding challenge. I am going to count the building of this app as around 10 days of coding, so I’m resuming the regular posts at Day 70 🙂.
I'm also accelerating my content creation work! TikTok, YouTube, and Twitter will all be seeing action in the short-to-mid-term. Some exciting stuff coming soon.
Very excited to get moving again. As always, thanks for all the support ♥️
Onwards!
Member discussion